Create the SocketIO Client
Video Lecture
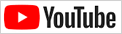
Description
First create a new HTML file called index.html in the ./dist/client/
folder and add this HTML.
./dist/client/index.html
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26 | <!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>
Socket.IO TypeScript Tutorials by Sean Bradley : https://sbcode.net/tssock/
</title>
<style>
body {
font-size: 4vw;
}
</style>
</head>
<body>
<script src="socket.io/socket.io.js"></script>
<script>
const socket = io()
socket.on('connect', () => {
document.body.innerText = 'connected : ' + socket.id
})
</script>
</body>
</html>
|
In order for our server to serve this static HTML file, we can use express
and point it to a folder to serve files from.
./src/server/server.ts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21 | import { createServer } from 'http'
import { Server } from 'socket.io'
import * as express from 'express'
import * as path from 'path'
const port = 3000
const app = express()
app.use(express.static(path.join(__dirname, '../client')))
const server = createServer(app)
const io = new Server(server)
io.on('connection', (socket) => {
console.log('a user connected : ' + socket.id)
})
server.listen(port, () => {
console.log('Server listening on port ' + port)
})
|
Install the Express module.
Also install the type declarations/definitions since they are not included with the express library.
npm install @types/express --save-dev
Restart the server,
Visit http://127.0.0.1:3000