Create the SocketIO Client
Video Lecture
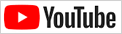
Description
First create a html file called index.html in the dist/client folder and add this html
./dist/client/index.html
1
2
3
4
5
6
7
8
9
10
11
12
13 | <!DOCTYPE html>
<html>
<head>
<title>TypeScript Socket.IO Course</title>
</head>
<body>
<script src="socket.io/socket.io.js"></script>
<script>
var socket = io()
</script>
</body>
</html>
|
Install express and it's types
npm install express@4
npm install @types/express@4 --save-dev
./src/server/server.ts
Note
In the video, my server.ts
is using Socket.IO 2.3.0
, the code below has now been updated to support Socket.IO 4.5.1
which means that line 19 has also been updated from
const io = socketIO(this.server)
to
const io = new socketIO.Server(this.server);
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29 | import express from 'express'
import path from 'path'
import http from 'http'
import socketIO from 'socket.io'
const port: number = 3000
class App {
private server: http.Server
private port: number
constructor(port: number) {
this.port = port
const app = express()
app.use(express.static(path.join(__dirname, '../client')))
this.server = new http.Server(app)
const io = new socketIO.Server(this.server)
}
public Start() {
this.server.listen(this.port, () => {
console.log(`Server listening on port ${this.port}.`)
})
}
}
new App(port).Start()
|
Visit http://127.0.0.1:3000
Useful Links
Server Options
https://socket.io/docs/v4/server-options/
Download Client JavaScript from a CDN
| <script
src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/3.0.4/socket.io.js"
integrity="sha512-aMGMvNYu8Ue4G+fHa359jcPb1u+ytAF+P2SCb+PxrjCdO3n3ZTxJ30zuH39rimUggmTwmh2u7wvQsDTHESnmfQ=="
crossorigin="anonymous"
></script>
|