Server Socket Broadcast
Video Lecture
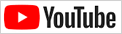
Description
Broadcast a message to all other connected sockets except for itself. Used most often after a socket level event occurs. E.g., a new message has arrived from an existing client socket, and you want to relay it onto all the other clients.
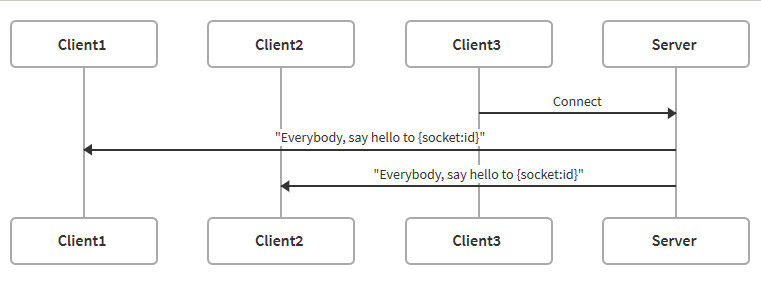
./src/server/server.ts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31 | import { createServer } from 'http'
import { Server } from 'socket.io'
import * as express from 'express'
import * as path from 'path'
const port = 3000
const app = express()
app.use(express.static(path.join(__dirname, '../client')))
const server = createServer(app)
const io = new Server(server)
io.on('connection', (socket) => {
console.log('a user connected : ' + socket.id)
socket.emit('message', 'Hello ' + socket.id)
socket.broadcast.emit('message', 'Everybody, say hello to ' + socket.id)
socket.on('disconnect', () => {
console.log('socket disconnected : ' + socket.id)
socket.broadcast.emit('message', socket.id + ' has left the building')
})
})
server.listen(port, () => {
console.log('Server listening on port ' + port)
})
|
./src/client/client.ts
| import { io } from 'socket.io-client'
const socket = io()
socket.on('connect', () => {
document.body.innerText = 'Connected : ' + socket.id
})
socket.on('message', (message) => {
document.body.innerHTML += '<p>' + message + '</p>'
})
|