Build an Example Mini Game
Video Lecture
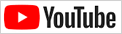
Description
We will now build this sample Mini Games server and client below using all of the things we've covered so far.
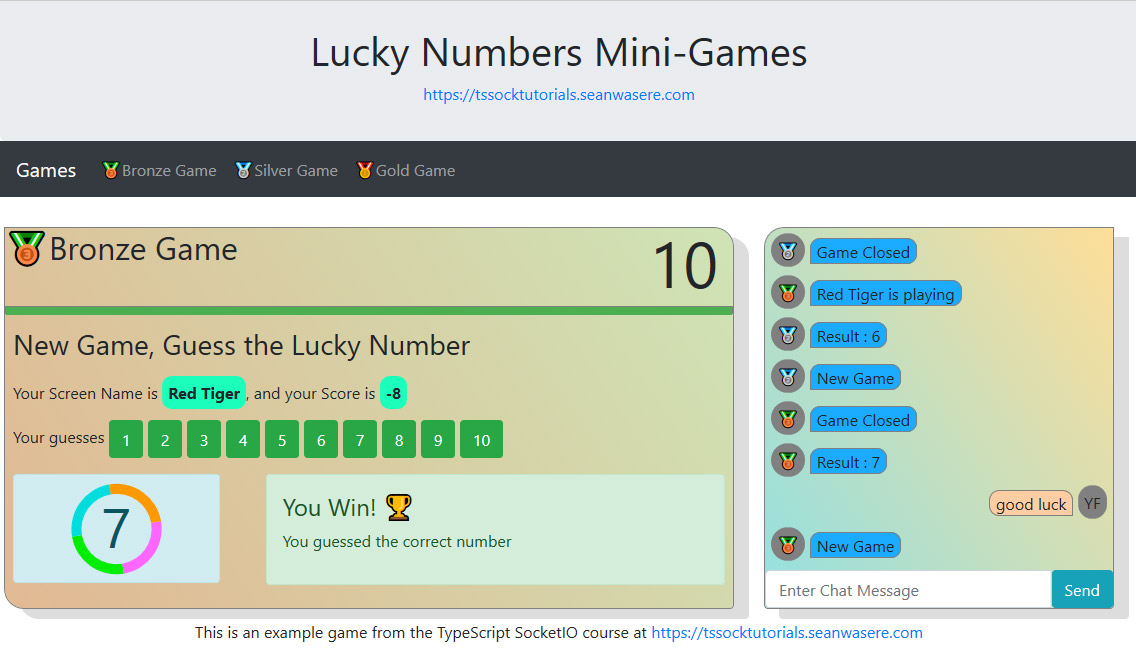
Before we start, ensure your folder structure is the same as below,
|-- tssocktutorial
|-- dist
|-- client
|-- server
|-- src
|-- client
|-- server
and your server.ts, client.ts, luckyNumbersGame.ts, tsconfigs, html and package.json scripts are also identical to below.
Note
A pre-built zip file containing the complete code and structure outlined in this page can be downloaded from the course resources on Udemy
./src/client/client.ts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18 | class Client {
private socket: SocketIOClient.Socket
constructor() {
this.socket = io()
this.socket.on('connect', function () {
console.log('connect')
})
this.socket.on('disconnect', function (message: any) {
console.log('disconnect ' + message)
location.reload()
})
}
}
const client = new Client()
|
./src/client/tsconfig.json
Note
In the videos i've targeted ES3
and module commonjs
for the browser. I now recommend using the below client tsconfig.json
that targets ES6 and ES6 modules.
1
2
3
4
5
6
7
8
9
10
11
12 | {
"compilerOptions": {
"target": "ES6",
"module": "ES6",
"outDir": "../../dist/client/",
"baseUrl": ".",
"paths": {},
"moduleResolution": "node",
"strict": true
},
"include": ["**/*.ts"]
}
|
./src/server/server.ts
Note
In the video, my server.ts
is using Socket.IO 2.3.0
, it now uses Socket.IO 4.5.1
which means that line 23 has now been updated from
this.io = socketIO(this.server)
to
this.io = new socketIO.Server(this.server);
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42 | import express from 'express'
import path from 'path'
import http from 'http'
import socketIO from 'socket.io'
import LuckyNumbersGame from './luckyNumbersGame'
const port: number = 3000
class App {
private server: http.Server
private port: number
private io: socketIO.Server
private game: LuckyNumbersGame
constructor(port: number) {
this.port = port
const app = express()
app.use(express.static(path.join(__dirname, '../client')))
this.server = new http.Server(app)
this.io = new socketIO.Server(this.server)
this.game = new LuckyNumbersGame()
this.io.on('connection', (socket: socketIO.Socket) => {
console.log('a user connected : ' + socket.id)
socket.on('disconnect', function () {
console.log('socket disconnected : ' + socket.id)
})
})
}
public Start() {
this.server.listen(this.port)
console.log(`Server listening on port ${this.port}.`)
}
}
new App(port).Start()
|
./src/server/luckyNumbersGame.ts
| export default class LuckyNumbersGame {
constructor() {}
}
|
./src/server/tsconfig.json
| {
"compilerOptions": {
"target": "ES2019",
"module": "commonjs",
"outDir": "../../dist/server",
"sourceMap": true,
"esModuleInterop": true
},
"include": ["**/*.ts"]
}
|
./dist/client/index.html
| <!DOCTYPE html>
<html>
<head>
<title>TypeScript Socket.IO Course</title>
</head>
<body>
<script src="socket.io/socket.io.js"></script>
<script src="client.js"></script>
</body>
</html>
|
./package.json
Note
The videos were created using Socket.IO 2.3.0
. The below package.json
and remaining course code examples now target Socket.IO 4.5.1
and Socket.IO-Client 4.5.1
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24 | {
"name": "tssocktutorial",
"version": "1.0.0",
"description": "",
"scripts": {
"dev": "concurrently -k \"tsc -p ./src/server -w\" \"tsc -p ./src/client -w\" \"nodemon ./dist/server/server.js\"",
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "Sean Bradley",
"license": "ISC",
"dependencies": {
"express": "^4.18.1",
"socket.io": "^4.5.1",
"socket.io-client": "^4.5.1"
},
"devDependencies": {
"@types/express": "^4.17.13",
"@types/node": "^18.0.4",
"@types/socket.io-client": "^1.4.36",
"concurrently": "^7.2.2",
"nodemon": "^2.0.19",
"typescript": "^4.7.4"
}
}
|
Run
And be sure you can successfully start it using
Warning
If npm run dev
fails to start, try building each of the TypeScript sub-projects individually first, then retry npm run dev
. E.g.,
tsc -p ./src/server
tsc -p ./src/client
npm run dev
Then visit http://127.0.0.1:3000