Install Bootstrap
Video Lecture
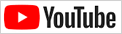
Description
We will use Bootstrap to help with the client interface layout, so we should install jQuery and Bootstrap@4.
npm install jquery@3
npm install @types/jquery@3 --save-dev
npm install bootstrap@4
npm install @types/bootstrap@4 --save-dev
Note
Be sure to install Bootstrap 4 as as shown in the above install command, since the course scripts have not yet been updated for Bootstrap 5.
./dist/client/index.html
HTML including the jQuery and Bootstrap dependencies
1
2
3
4
5
6
7
8
9
10
11
12
13
14 | <!DOCTYPE html>
<html>
<head>
<title>TypeScript Socket.IO Course</title>
<link rel="stylesheet" href="bootstrap/css/bootstrap.min.css" />
</head>
<body>
<script src="jquery/jquery.min.js"></script>
<script src="bootstrap/js/bootstrap.bundle.min.js"></script>
<script src="socket.io/socket.io.js"></script>
<script src="client.js"></script>
</body>
</html>
|
./src/server/server.ts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54 | import express from 'express'
import path from 'path'
import http from 'http'
import socketIO from 'socket.io'
import LuckyNumbersGame from './luckyNumbersGame'
const port: number = 3000
class App {
private server: http.Server
private port: number
private io: socketIO.Server
private game: LuckyNumbersGame
constructor(port: number) {
this.port = port
const app = express()
app.use(express.static(path.join(__dirname, '../client')))
app.use(
'/jquery',
express.static(
path.join(__dirname, '../../node_modules/jquery/dist')
)
)
app.use(
'/bootstrap',
express.static(
path.join(__dirname, '../../node_modules/bootstrap/dist')
)
)
this.server = new http.Server(app)
this.io = new socketIO.Server(this.server)
this.game = new LuckyNumbersGame()
this.io.on('connection', (socket: socketIO.Socket) => {
console.log('a user connected : ' + socket.id)
socket.on('disconnect', function () {
console.log('socket disconnected : ' + socket.id)
})
})
}
public Start() {
this.server.listen(this.port)
console.log(`Server listening on port ${this.port}.`)
}
}
new App(port).Start()
|