Materials
Video Lecture
Section |
Video Links |
|
Materials |
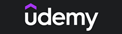 |
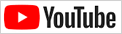 |
Description
In this lesson, we will introduce four of the most commonly used materials that you will see used in Three.js.
- MeshBasicMaterial
- MeshNormalMaterial
- MeshPhongMaterial
- MeshStandardMaterial
There are other materials, but we will discuss those, plus more details, about each material as we progress.
In this lesson, we will start by focusing on several important factors about materials and why you might choose one over the others.
./src/App.jsx
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36 | import { Canvas } from '@react-three/fiber'
import Polyhedron from './Polyhedron'
import * as THREE from 'three'
import { Stats, OrbitControls } from '@react-three/drei'
export default function App() {
return (
<Canvas camera={{ position: [-1, 4, 2.5] }}>
<directionalLight position={[1, 1, 1]} />
<Polyhedron
name="meshBasicMaterial"
position={[-3, 1, 0]}
material={new THREE.MeshBasicMaterial()}
/>
<Polyhedron
name="meshNormalMaterial"
position={[-1, 1, 0]}
material={new THREE.MeshNormalMaterial()}
/>
<Polyhedron
name="meshPhongMaterial"
position={[1, 1, 0]}
material={new THREE.MeshPhongMaterial()}
/>
<Polyhedron
name="meshStandardMaterial"
position={[3, 1, 0]}
material={new THREE.MeshStandardMaterial()}
/>
<OrbitControls target-y={1} />
<axesHelper args={[5]} />
<gridHelper />
<Stats />
</Canvas>
)
}
|
./src/Polyhedron.jsx
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41 | import { useRef } from 'react'
import { useControls } from 'leva'
import * as THREE from 'three'
import { useFrame } from '@react-three/fiber'
export default function Polyhedron(props) {
const ref = useRef()
useFrame((_, delta) => {
ref.current.rotation.x += 0.2 * delta
ref.current.rotation.y += 0.05 * delta
})
useControls(props.name, {
wireframe: {
value: false,
onChange: (v) => {
ref.current.material.wireframe = v
},
},
flatShading: {
value: true,
onChange: (v) => {
ref.current.material.flatShading = v
ref.current.material.needsUpdate = true
},
},
color: {
value: 'lime',
onChange: (v) => {
ref.current.material.color = new THREE.Color(v)
},
},
})
return (
<mesh {...props} ref={ref}>
<icosahedronGeometry args={[1, 1]} />
</mesh>
)
}
|
Working Example
Useful Links
GitHub Branch
git clone https://github.com/Sean-Bradley/React-Three-Fiber-Boilerplate.git
cd React-Three-Fiber-Boilerplate
git checkout materials
npm install
npm start