Server IO Emit
Video Lecture
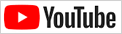
Description
Emit a message to all connected sockets.
Used most often when a server level event occurs, eg, a timer event occured and you want to send a message to all clients.
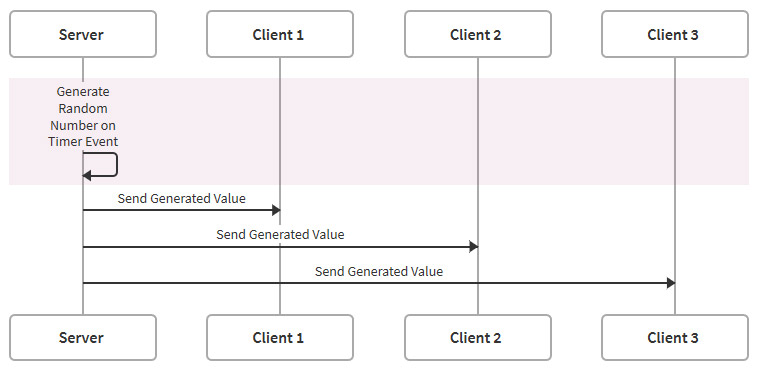
./src/server/server.ts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49 | import express from 'express'
import path from 'path'
import http from 'http'
import socketIO from 'socket.io'
const port: number = 3000
class App {
private server: http.Server
private port: number
private io: socketIO.Server
constructor(port: number) {
this.port = port
const app = express()
app.use(express.static(path.join(__dirname, '../client')))
this.server = new http.Server(app)
this.io = new socketIO.Server(this.server)
this.io.on('connection', (socket: socketIO.Socket) => {
console.log('a user connected : ' + socket.id)
socket.emit('message', 'Hello ' + socket.id)
socket.broadcast.emit(
'message',
'Everybody, say hello to ' + socket.id
)
socket.on('disconnect', function () {
console.log('socket disconnected : ' + socket.id)
})
})
setInterval(() => {
this.io.emit('random', Math.floor(Math.random() * 10))
}, 1000)
}
public Start() {
this.server.listen(this.port)
console.log(`Server listening on port ${this.port}.`)
}
}
new App(port).Start()
|
./src/client/client.ts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19 | class Client {
private socket: SocketIOClient.Socket
constructor() {
this.socket = io()
this.socket.on('message', function (message: any) {
console.log(message)
document.body.innerHTML += message + '<br/>'
})
this.socket.on('random', function (message: any) {
console.log(message)
document.body.innerHTML += 'Winning number is ' + message + '<br/>'
})
}
}
const client = new Client()
|