GLTF Animations
Video Lecture
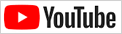
Description
In this lesson, we will use GLTF animations both stored in the GLTF and external.
We will use Blender to convert FBX models and animations from Mixamo, into GLB files.
Resources
The 3D model and animations used in this lesson can easily be downloaded from Mixamo and converted using Blender. If you don't want to download and convert, then you can download the converted files using the link below. Extract the contents of the zip file into your ./public/models/
folder.
gltf-animations.zip
Lesson Script
./src/main.ts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140 | import './style.css'
import * as THREE from 'three'
import { OrbitControls } from 'three/addons/controls/OrbitControls.js'
import { RGBELoader } from 'three/addons/loaders/RGBELoader.js'
import { GLTFLoader } from 'three/addons/loaders/GLTFLoader.js'
import Stats from 'three/addons/libs/stats.module.js'
const scene = new THREE.Scene()
const gridHelper = new THREE.GridHelper(100, 100)
scene.add(gridHelper)
new RGBELoader().load('img/venice_sunset_1k.hdr', (texture) => {
texture.mapping = THREE.EquirectangularReflectionMapping
scene.environment = texture
scene.background = texture
scene.backgroundBlurriness = 1
})
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 100)
camera.position.set(0.1, 1, 1)
const renderer = new THREE.WebGLRenderer({ antialias: true })
renderer.setSize(window.innerWidth, window.innerHeight)
document.body.appendChild(renderer.domElement)
window.addEventListener('resize', () => {
camera.aspect = window.innerWidth / window.innerHeight
camera.updateProjectionMatrix()
renderer.setSize(window.innerWidth, window.innerHeight)
})
const controls = new OrbitControls(camera, renderer.domElement)
controls.enableDamping = true
controls.target.set(0, 0.75, 0)
const stats = new Stats()
document.body.appendChild(stats.dom)
// function lerp(from: number, to: number, speed: number) {
// const amount = (1 - speed) * from + speed * to
// return Math.abs(from - to) < 0.001 ? to : amount
// }
//let mixer: THREE.AnimationMixer
//let animationActions: { [key: string]: THREE.AnimationAction } = {}
//let activeAction: THREE.AnimationAction
// let speed = 0,
// toSpeed = 0
new GLTFLoader().load('models/eve$@walk.glb', (gltf) => {
// mixer = new THREE.AnimationMixer(gltf.scene)
// //console.log(gltf )
// mixer.clipAction(gltf.animations[0]).play()
scene.add(gltf.scene)
})
// async function loadEve() {
// const loader = new GLTFLoader()
// const [eve, idle, run] = await Promise.all([
// loader.loadAsync('models/eve$@walk.glb'),
// loader.loadAsync('models/eve@idle.glb'),
// loader.loadAsync('models/eve@run.glb')
// ])
// mixer = new THREE.AnimationMixer(eve.scene)
// // mixer.clipAction(idle.animations[0]).play()
// // animationActions['idle'] = mixer.clipAction(idle.animations[0])
// // animationActions['walk'] = mixer.clipAction(eve.animations[0])
// // animationActions['run'] = mixer.clipAction(run.animations[0])
// // animationActions['idle'].play()
// // activeAction = animationActions['idle']
// scene.add(eve.scene)
// }
// await loadEve()
// const keyMap: { [key: string]: boolean } = {}
// const onDocumentKey = (e: KeyboardEvent) => {
// keyMap[e.code] = e.type === 'keydown'
// }
// document.addEventListener('keydown', onDocumentKey, false)
// document.addEventListener('keyup', onDocumentKey, false)
// const clock = new THREE.Clock()
// let delta = 0
function animate() {
requestAnimationFrame(animate)
//delta = clock.getDelta()
controls.update()
//mixer.update(delta)
// if (keyMap['KeyW']) {
// if (keyMap['ShiftLeft']) {
// //run
// if (activeAction != animationActions['run']) {
// activeAction.fadeOut(0.5)
// animationActions['run'].reset().fadeIn(0.25).play()
// activeAction = animationActions['run']
// // toSpeed = 4
// }
// } else {
// //walk
// if (activeAction != animationActions['walk']) {
// activeAction.fadeOut(0.5)
// animationActions['walk'].reset().fadeIn(0.25).play()
// activeAction = animationActions['walk']
// // toSpeed = 1
// }
// }
// } else {
// //idle
// if (activeAction != animationActions['idle']) {
// activeAction.fadeOut(0.5)
// animationActions['idle'].reset().fadeIn(0.25).play()
// activeAction = animationActions['idle']
// // toSpeed = 0
// }
// }
// speed = lerp(speed, toSpeed, delta * 10)
// gridHelper.position.z -= speed * delta
// gridHelper.position.z = gridHelper.position.z % 10
renderer.render(scene, camera)
stats.update()
}
animate()
|
Useful Links
Blender
Mixamo
https://threejs.org/docs/#api/en/animation/AnimationMixer
https://threejs.org/docs/#api/en/animation/KeyframeTrack